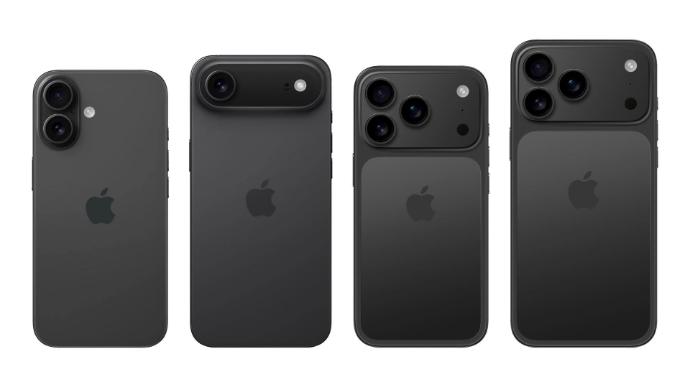
iPhone 17 Pro Max Price & Release Date
1. “iPhone 17 Pro Max Price & Release Date: What to Expect” Introduction Apple’s iPhone 17 Pro Max is one of the most anticipated smartphones of 2025, with rumors swirling...
Continue reading
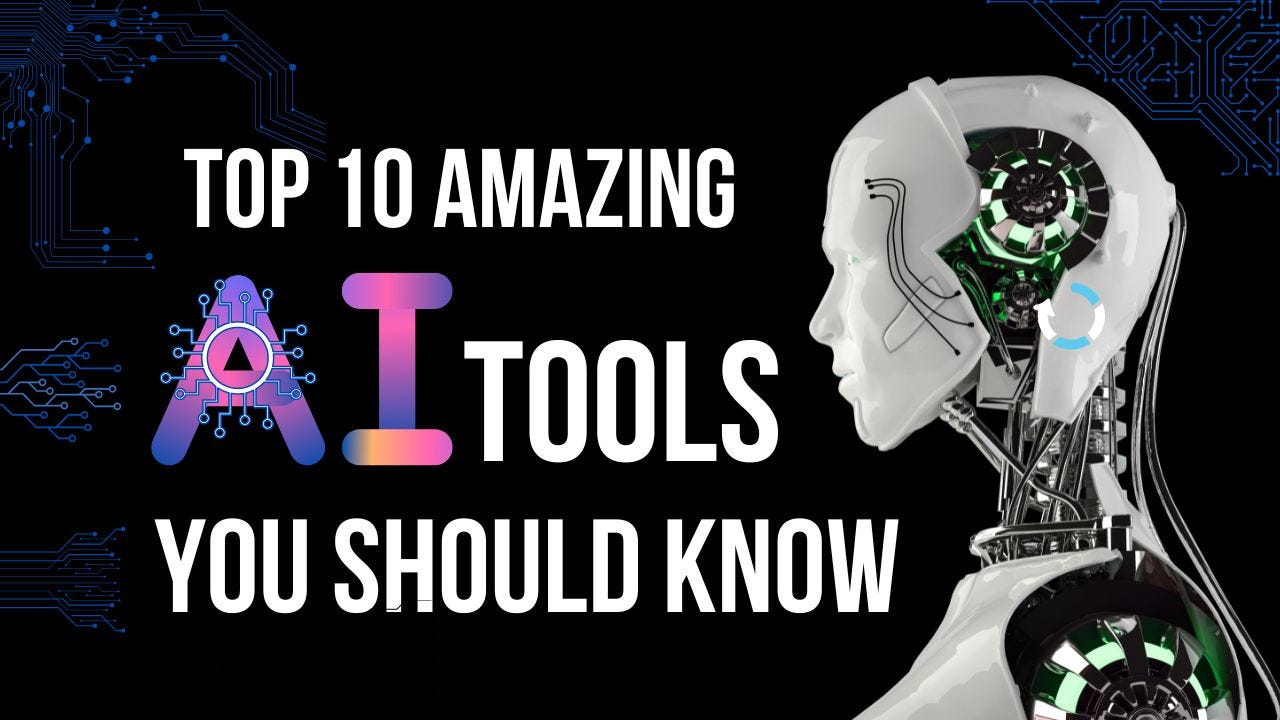
The Hidden Gems: 7 Underrated Free AI Tools You Should Try Today
Introduction: Beyond ChatGPT – Discover Powerful, Lesser-Known AI While everyone’s using ChatGPT and Midjourney, dozens of highly capable AI tools fly under the radar. These hidden gems offer unique capabilities...
Continue reading
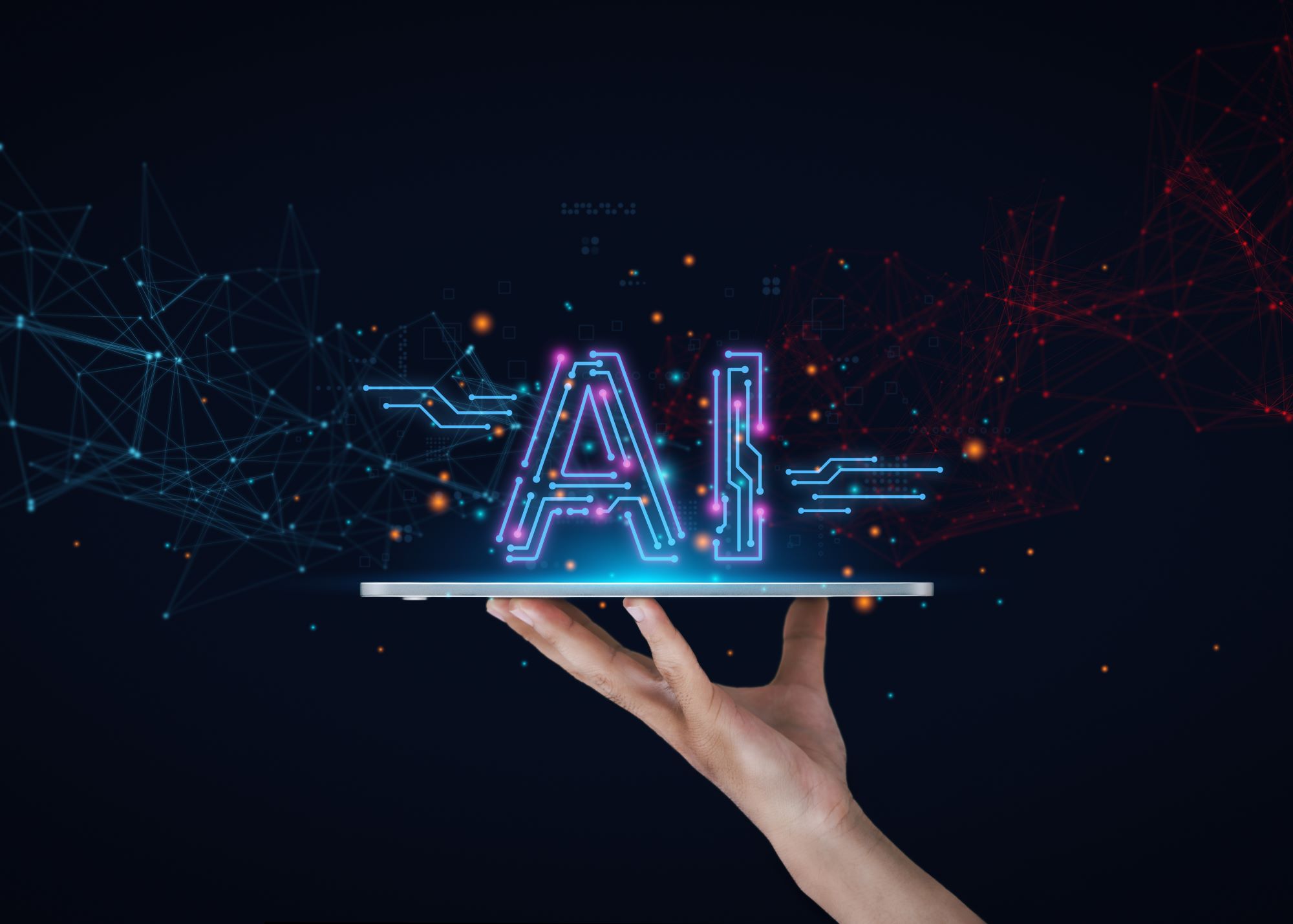
Free AI Tools for Students: Ace Homework, Research & Time Management
Introduction: AI as Your 24/7 Study Partner Between lectures, assignments, and exams, student life is overwhelming. But what if you had an AI-powered toolkit to research faster, write better, and...
Continue reading
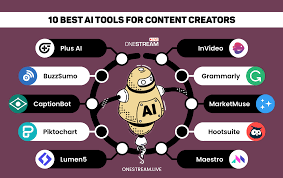
5 Best Free AI Tools for Content Creators (Bloggers, YouTubers & Social Media Managers)
Introduction: The AI Advantage for Content Creation Content creation is more competitive than ever. Whether you’re a blogger, YouTuber, or social media manager, AI tools can help you work faster,...
Continue reading
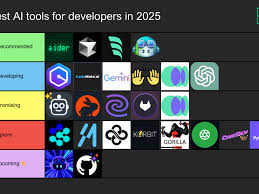
Got it! Below is a detailed, long-form blog post for your first topic. If you like the format, I’ll proceed with the remaining four. Let me know any adjustments you’d prefer (tone, depth, structure, etc.).
10 Free AI Tools to Supercharge Your Productivity in 2024 Introduction: Why AI Tools Are a Game-Changer In 2024, AI isn’t just a luxury—it’s a necessity for staying competitive. From...
Continue reading
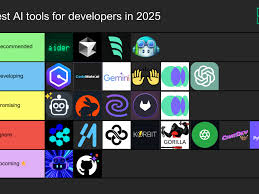
Here are five engaging blog post ideas about free AI tools that can help users boost productivity, creativity, and efficiency:
1. “10 Free AI Tools to Supercharge Your Productivity in 2024” 2. “5 Best Free AI Tools for Content Creators (Bloggers, YouTubers & Social Media Managers)” 3. “How to Use...
Continue reading
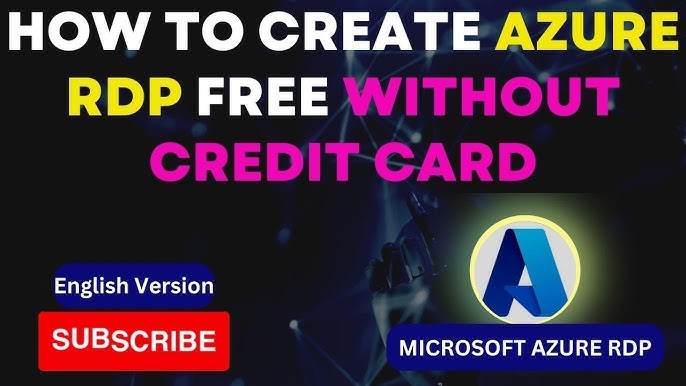
How to Get Free Windows 11 RDP on Azure Without Licensing Issues (2025)
The Windows Licensing Challenge One of the biggest hurdles when setting up a free Azure RDP is Windows licensing costs. While Linux VMs are completely free, Microsoft charges for Windows...
Continue reading
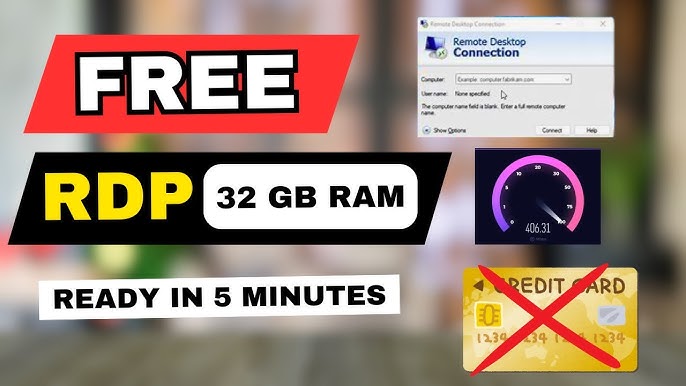
Top 5 Legit Ways to Get Free Azure RDP for Developers & Students (2025)
Introduction Getting a free Azure RDP in 2025 is still possible if you know where to look. Many students and developers need cloud resources for projects, coding, or testing –...
Continue reading
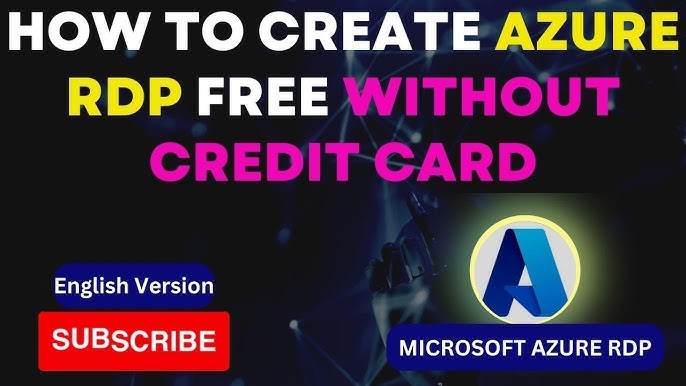
Is Microsoft Azure RDP Really Free? Understanding the Limits (2025)
Introduction Many users search for “Free Azure RDP”, but is it truly free? While Microsoft Azure offers free-tier services, there are hidden limitations and potential costs that can catch users...
Continue reading
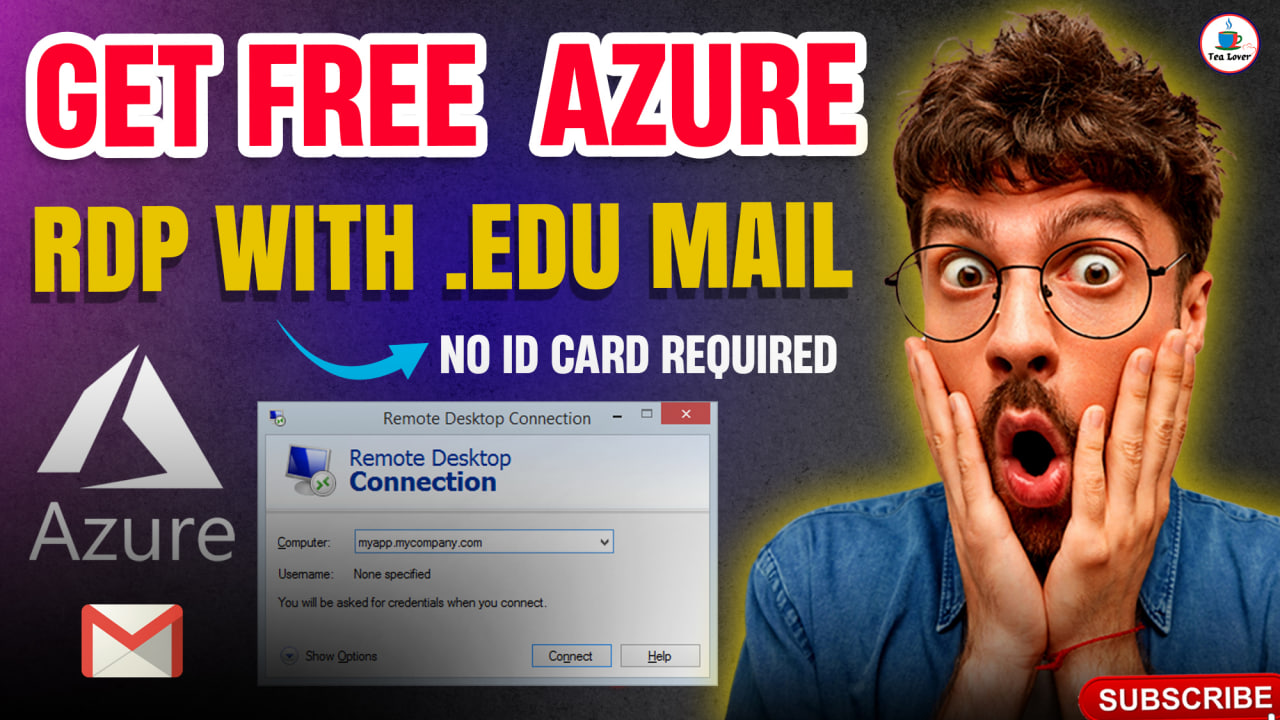
How to Get a Free Azure RDP in 2025 – Step-by-Step Guide
Introduction Remote Desktop Protocol (RDP) allows users to access a remote computer over the internet. Microsoft Azure offers cloud-based RDP solutions, and in 2025, there are still ways to get...
Continue reading